Small Find Diff example#
import pyomo.environ as pyo
numpoints = 5
model = m = pyo.ConcreteModel()
m.points = pyo.RangeSet(0,numpoints-1)
m.h = pyo.Param(initialize=1.0/(numpoints-1))
m.z = pyo.Var(m.points)
m.dzdt = pyo.Var(m.points)
m.obj = pyo.Objective(expr=1) # Dummy Objective
def _zdot(m, i):
return m.dzdt[i] == m.z[i]**2 - 2*m.z[i] +1
m.zdot = pyo.Constraint(m.points,rule=_zdot)
def _back_diff(m,i):
if i == 0:
return pyo.Constraint.Skip
return m.dzdt[i] == (m.z[i]-m.z[i-1])/m.h
m.back_diff = pyo.Constraint(m.points,rule=_back_diff)
def _init_con(m):
return m.z[0] == -3
m.init_con = pyo.Constraint(rule=_init_con)
solver = pyo.SolverFactory('ipopt')
solver.solve(m,tee=True)
import matplotlib.pyplot as plt
analytical_t = [0.01*i for i in range(0,101)]
analytical_z = [(4*t-3)/(4*t+1) for t in analytical_t]
findiff_t = [m.h*i for i in m.points]
findiff_z = [pyo.value(m.z[i]) for i in m.points]
plt.plot(analytical_t,analytical_z,'b',label='analytical solution')
plt.plot(findiff_t,findiff_z,'ro--',label='finite difference solution')
plt.legend(loc='best')
plt.xlabel('t')
plt.show()
Ipopt 3.13.2:
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma27.
Number of nonzeros in equality constraint Jacobian...: 23
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 5
Total number of variables............................: 10
variables with only lower bounds: 0
variables with lower and upper bounds: 0
variables with only upper bounds: 0
Total number of equality constraints.................: 10
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 1.0000000e+00 3.00e+00 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 1.0000000e+00 1.74e+00 0.00e+00 -1.0 7.00e+00 - 1.00e+00 1.00e+00H 1
2 1.0000000e+00 5.79e-02 0.00e+00 -1.0 8.14e-01 - 1.00e+00 1.00e+00h 1
3 1.0000000e+00 1.47e-04 0.00e+00 -2.5 2.24e-02 - 1.00e+00 1.00e+00h 1
4 1.0000000e+00 1.73e-09 0.00e+00 -5.7 6.47e-05 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 4
(scaled) (unscaled)
Objective...............: 1.0000000000000000e+00 1.0000000000000000e+00
Dual infeasibility......: 0.0000000000000000e+00 0.0000000000000000e+00
Constraint violation....: 1.7255730178078466e-09 1.7255730178078466e-09
Complementarity.........: 0.0000000000000000e+00 0.0000000000000000e+00
Overall NLP error.......: 1.7255730178078466e-09 1.7255730178078466e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 5
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 5
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 4
Total CPU secs in IPOPT (w/o function evaluations) = 0.003
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
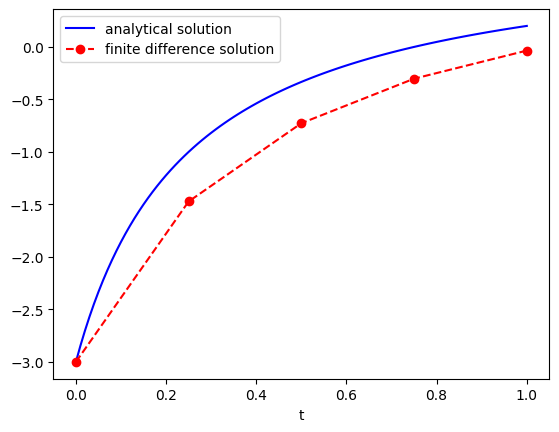