Small Dae Example#
import pyomo.environ as pyo
from pyomo.dae import DerivativeVar, ContinuousSet
model = m = pyo.ConcreteModel()
m.t = ContinuousSet(bounds=(0,1))
m.z = pyo.Var(m.t)
m.dzdt = DerivativeVar(m.z)
m.obj = pyo.Objective(expr=1) # Dummy Objective
def _zdot(m, i):
return m.dzdt[i] == m.z[i]**2 - 2*m.z[i] +1
m.zdot = pyo.Constraint(m.t,rule=_zdot)
def _init_con(m):
return m.z[0] == -3
m.init_con = pyo.Constraint(rule=_init_con)
# Discretize using backward finite difference
#discretizer = pyo.TransformationFactory('dae.finite_difference')
#discretizer.apply_to(m, nfe=50, scheme='BACKWARD')
# Discretize using collocation
discretizer = pyo.TransformationFactory('dae.collocation')
discretizer.apply_to(m, nfe=2, ncp=3 , scheme='LAGRANGE-RADAU')
solver = pyo.SolverFactory('ipopt')
solver.solve(m,tee=True)
import matplotlib.pyplot as plt
analytical_t = [0.01*i for i in range(0,101)]
analytical_z = [(4*t-3)/(4*t+1) for t in analytical_t]
findiff_t = list(m.t)
findiff_z = [pyo.value(m.z[i]) for i in m.t]
plt.plot(analytical_t,analytical_z,'b',label='analytical solution')
plt.plot(findiff_t,findiff_z,'ro--',label='pyomo.dae discretization solution')
plt.legend(loc='best')
plt.xlabel('t')
plt.show()
Ipopt 3.14.17:
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
This is Ipopt version 3.14.17, running with linear solver MUMPS 5.7.3.
Number of nonzeros in equality constraint Jacobian...: 45
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 7
Total number of variables............................: 14
variables with only lower bounds: 0
variables with lower and upper bounds: 0
variables with only upper bounds: 0
Total number of equality constraints.................: 14
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 1.0000000e+00 3.00e+00 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 1.0000000e+00 2.29e+00 0.00e+00 -1.0 7.00e+00 - 1.00e+00 1.00e+00H 1
2 1.0000000e+00 1.92e-01 0.00e+00 -1.0 1.53e+00 - 1.00e+00 1.00e+00h 1
3 1.0000000e+00 2.37e-03 0.00e+00 -2.5 1.02e-01 - 1.00e+00 1.00e+00h 1
4 1.0000000e+00 5.51e-07 0.00e+00 -3.8 1.37e-03 - 1.00e+00 1.00e+00h 1
5 1.0000000e+00 7.77e-15 0.00e+00 -8.6 4.11e-07 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 1.0000000000000000e+00 1.0000000000000000e+00
Dual infeasibility......: 0.0000000000000000e+00 0.0000000000000000e+00
Constraint violation....: 7.7715611723760958e-15 7.7715611723760958e-15
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 0.0000000000000000e+00 0.0000000000000000e+00
Overall NLP error.......: 7.7715611723760958e-15 7.7715611723760958e-15
Number of objective function evaluations = 7
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total seconds in IPOPT = 0.003
EXIT: Optimal Solution Found.
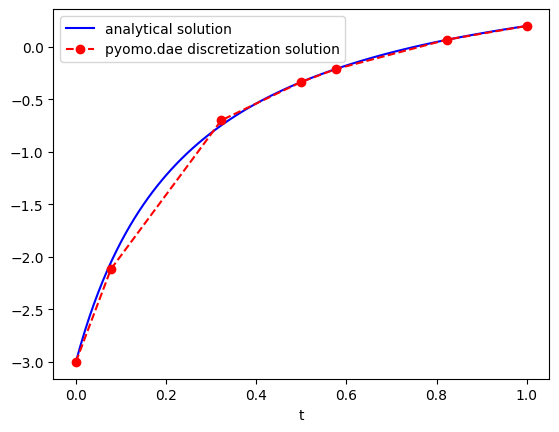